Web scraping is the process of extracting data from websites. This can be useful for gathering large amounts of data for analysis. JavaScript is a popular language for web scraping due to its built-in DOM manipulation capabilities and ubiquity in web browsers. ChatGPT is an AI assistant that can be helpful for generating code and explanations for web scraping tasks. This article will provide an overview of web scraping in JavaScript and how ChatGPT can assist.
Setting Up a JavaScript Environment
To use JavaScript for web scraping, you'll need a JavaScript runtime like Node.js installed on your system. You'll also need to install JavaScript libraries like request for sending HTTP requests and cheerio for parsing HTML.
// Install request
npm install request
// Install cheerio
npm install cheerio
Introduction to Web Scraping
Web scraping involves programmatically fetching data from websites. This is done by sending HTTP requests to the target site and parsing the HTML, XML or JSON response. Popular JavaScript libraries for web scraping include:
The general workflow for a basic web scraper is:
This can be extended to scrape various data types, handle pagination, scrape JavaScript generated content, avoid detection etc.
ChatGPT for Web Scraping Help
ChatGPT is an AI assistant created by OpenAI to be helpful, harmless, and honest. It can generate natural language explanations and code for a variety of topics. For web scraping, some ways ChatGPT can help are:
Generating Explanations
If you are stuck on a web scraping task, ChatGPT can provide explanations of web scraping concepts or specifics for your use case. Some examples:
Writing Code Snippets
You can provide a description of what you want your code to do and have ChatGPT generate starter code snippets for you. For example:
Be sure to validate any code ChatGPT provides before using it.
Improving Your Prompts
If ChatGPT is not providing helpful responses, you can ask it to suggest ways to improve your prompt to get better results.
Asking Follow-up Questions
Engage in a back and forth conversation with ChatGPT to get explanations for any follow-up questions you have.
Explaining Errors
Share any errors you are getting and ask ChatGPT to explain the issue and how to fix it.
Web Scraping Example Using ChatGPT
Let's go through an example of web scraping a Wikipedia page with some help from ChatGPT along the way.
Goal
The goal is to get the chronology of the universe located in this Wikipedia page https://en.wikipedia.org/wiki/Chronology_of_the_universe
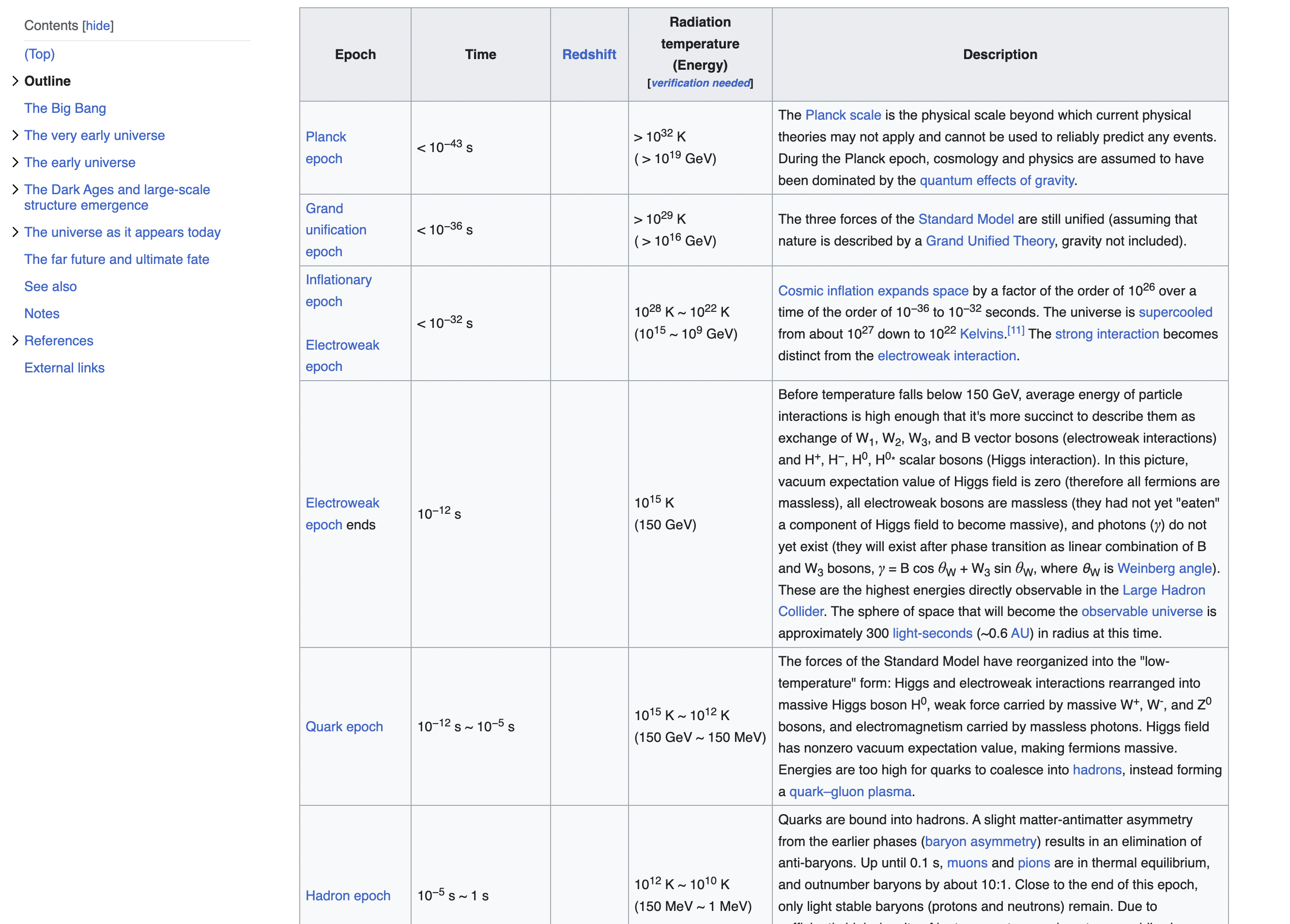
There is a nice table in the page that gives you the timeline of events and various epochs...
Step 1: The first task is to download the page. Lets ask ChatGPT for help here with this prompt...
give me a script to download this page in javascript... <https://en.wikipedia.org/wiki/Chronology_of_the_universe>
// ChatGPT should give you this code
const request = require('request');
const url = '<https://en.wikipedia.org/wiki/Chronology_of_the_universe>';
request(url, (error, response, body) => {
if(error) {
console.error('Error:', error);
} else {
// Save response to file
const fs = require('fs');
fs.writeFile('chronology.html', body, (err) => {
if(err) console.error(err);
else console.log('Page downloaded successfully');
});
}
});
Step 2: Inspect the HTML and look for hints that we can pass to chatGPT. In this case, you can see that the whole table has a class named wikitable
Step 3: Help out ChatGPT with this info. Here is the prompt
I want the script to extract a table with the class wikitable - The
// ChatGPT should return the following code
const request = require('request');
const cheerio = require('cheerio');
const fs = require('fs');
const url = '<https://en.wikipedia.org/wiki/Chronology_of_the_universe>';
request(url, (error, response, html) => {
if (!error){
const $ = cheerio.load(html);
const table = $('.wikitable');
const headers = [];
table.find('th').each(function(){
headers.push($(this).text());
});
const rows = [];
table.find('tr').each(function(){
const row = [];
$(this).find('td').each(function(){
row.push($(this).text());
});
rows.push(row);
});
fs.writeFileSync('wikitable.csv', headers.join(',') + '\\n' + rows.map(row => row.join(',')).join('\\n'));
console.log('Extracted table to wikitable.csv');
} else {
console.error(error);
}
});
And this saves the extracted data to a CSV file.
This demonstrates how ChatGPT can rapidly generate web scraping code snippets in JavaScript once you provide some hints about the page structure and data you want to extract. The conversational prompting works for Python, PHP, JS or any language.
Conclusion
Some key points:
With JavaScript and some help from ChatGPT, you can quickly build scrapers to extract information from websites.
ChatGPT heralds an exciting new era in intelligent automation!
However, this approach also has some limitations:
A more robust solution is using a dedicated web scraping API like Proxies API
With Proxies API, you get:
With features like automatic IP rotation, user-agent rotation and CAPTCHA solving, Proxies API makes robust web scraping easy via a simple API:
fetch("<https://api.proxiesapi.com/?key=API_KEY&url=targetsite.com>")
.then(response => response.json())
.then(data => {
// Use scraped data
});
Get started now with 1000 free API calls to supercharge your web scraping!
Browse by tags:
Browse by language:
The easiest way to do Web Scraping
Get HTML from any page with a simple API call. We handle proxy rotation, browser identities, automatic retries, CAPTCHAs, JavaScript rendering, etc automatically for you
Try ProxiesAPI for free
curl "http://api.proxiesapi.com/?key=API_KEY&url=https://example.com"
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
...
Don't leave just yet!
Enter your email below to claim your free API key: